Lesson 9 - Right_or_Wrong.py
In this lesson, we are going to learn how to take the System_Check.py script and turn it into a new program with a correct button and an incorrect button. The good news: it only takes ctrl + c, ctrl + p, and some slight modifications to the code.
Program Structure
As always, before we even begin writing code, it is important to start with our program structure. Here is a video of what we want to accomplish:
Here is a visual diagram of what we want the program to do:
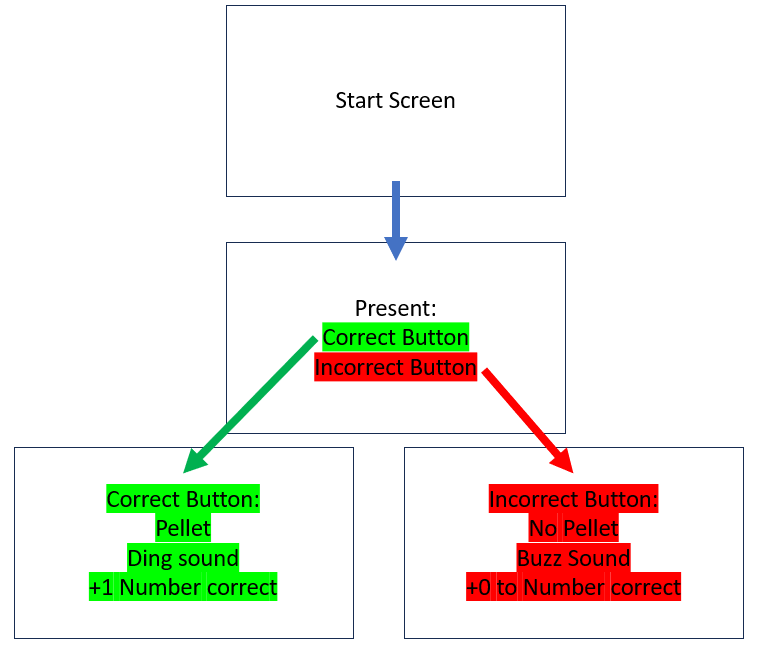
Include a third stimuli
This is already built into the program file and system_check.py; however, if it wasn’t you would need to add in a third stimuli. Now you have the start button, the correct button, and the incorrect button.
def create_stimuli(self):
Icons = [Icon("start.png", (150, 200), (140, 140)),
Icon("correct.png", (0, 0), (100, 100)),
Icon("incorrect.png", (0, 0), (100, 100))
]
self.stimuli = [Icons[0], Icons[1], Icons[2]]
Draw both buttons at different locations
Once you have both stimuli in the list “self.stimuli” we need to draw them. Make sure they are drawn at different locations, otherwise you won’t see because they are on top of each other. We do this by choosing another position in the self.icon_position[] list.
def draw_stimuli(self):
self.stimuli[1].mv2pos(self.icon_position[0])
self.stimuli[1].draw(screen)
self.stimuli[2].mv2pos(self.icon_position[1])
self.stimuli[2].draw(screen)
Tell the program what to do if the incorrect button is selected
Now your program draws the buttons, but if the cursor hits the red box, nothing happens. Next hop down to the run_trial(self) function and add an else-if statement. If the cursor collides with the red box, write 0 in the data file, play the buzz sound, and start the next trial.
elif cursor.collides_with_list(self.stimuli) == 2:
self.write(data_file, 0)
sound(False)
screen.fill(white)
refresh(screen)
pygame.time.delay(time_out * 1000)
self.new()